Hardware
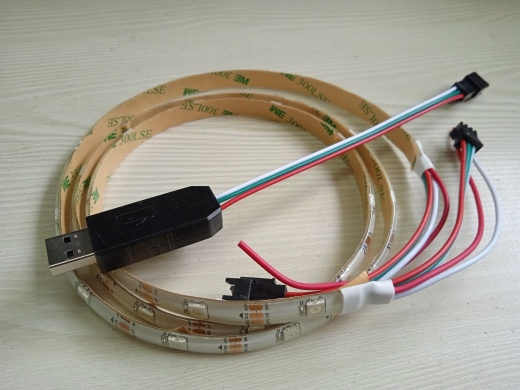
Shell Lab L2 is programmable LED strip (WS2812 chip) with USB/serial interface Characteristic: ● supports WS2812 LED chip, 5V powered● configurable strip length ● signal lamp mode: whole strip treated as same color, color/frequency configurable ● RGB each channel is independently adjusted from 0~255 ● blinking effect (freq adjustable from 0.1~20Hz, 0 for static) ● addressable mode: assign color for each single LED chip ● interactive serial console with ASCII command, online manual embedded ● programming language is not limited (Python recommended) ● quick test with Testbench App (with various demo codes) ● pip install mcush library (support windows/linux/mac) and write scripts easily Use cases: ● prototype design● fault/exception indication in product/software cycle test ● indication in assembly line, green for PASS and red for FAIL ● experiment progress indication ● kanban/placard indication ● logistic/storage management, goods location indicator ● ROS robot indication ● interactive game design ● educational experiment design ● outdoor case, auto indicator ● system integration for industrial equipment ● art creativity ● DIY toy ● shop sign for advertisement Work modes: ● signal lamp mode (all LEDs blink in same color and frequency)● addressable mode (each LED is configurable with its own static color) Customize: ● customize PCB and case● customize firmware for module integration ● switch to bluetooth communication, for mobile control ● RS232 interfaced ![]() ● Multi strips controller ![]() ● customized for outdoor use (16 chips serialized internally) ![]() Attention: ● commands in lamp mode are very similar to L1 signal lamp, easy for replacement● limited for USB power capacity, add extra 5V power for longer strip (at the strip tail) |
Software
Serial Communication FAQ C Programming FAQ Serial command: 1. Signal lamp mode
Example: After poweron, assign the actual strip length first:
Then send the color(basic colors): black: 0x000000 red: 0xFF0000 green: 0x00FF00 blue: 0x0000FF yellow: 0xFFFF00 cyan: 0x00FFFF purple: 0xFF00FF white: 0xFFFFFF blink in RED color, 1Hz(default):
blink in YELLOW color, 2Hz:
blink in BLUE color, 0.5Hz:
blink in YELLOW color, 0.1Hz:
blink in PURPLE color, 10Hz:
static CRAY color:
single RED channel as 64:
single GREEN channel as 0x80:
single BLUE channel as 0x200:
query current length/color/freq setting:
2. Addressable mode:
Example (standard mode): signal lamp mode must be disabled first (modes conflict), reset length to zero with strap command,then init length with ws2812 command: (note: limited to chip memory, actual length is no longer than 300)
all leds are allocated with independent memory and set zero/black, but not synced to strip, update them:
fill #3~5 leds with red/green/blue, sync and update:
fill #10~20 leds with purple, sync and update:
move whole leds forward, fill #0 led with blue, sync and update:
move whole leds backward, fill #29 led with green, sync and update:
move top half leds backward, fill #29 led with green, no sync,move bottom half leds forward, fill #0 led with green, sync together:
if leds pattern (in sequence of 0xRR,0xGG,0xBB 3bytes) has been saved into file (by script tool),the file can be quickly/directly loaded into strip, suitable for load preset patterns/bitmaps.
Example (group mode): combine continuous leds together into group, share the same memory,more leds can be controlled with same memory, total sync time will be longer. 30 leds split into 10 groups (3 leds in each group), init:
fill whole strip in pattern green/blue alternatively:
Example (resizeable group length): practically, for assembly reason, assign groups with different length may be more effectivegroup list length needs to be defined into config file and written into flash (script tool needed) if /c/glist has been written, contains binary defination0x03,0x05,0x0A.. for each group length, init:
Python API: Install: sudo pip3 install mcushUpgrade: sudo pip3 install -U mcush
Examples:
Download: Shell Lab Testbench Application |
Application
Blink effect in signal lamp mode
Multi color blinking
Long strip
Use push command
Push command (faster)
Push command (32*8=256 LEDs, rainbow waterfall)
Configured as RGBCMY loop after poweron download
Color heart lamp customized with the above code
Configured as police alarm flashdownload
Code effect
C-API Compiled waterfall
Effect for the above code
Configured as a command-triggered waterfall stripdownload
Effect for the above code
Configured to switch between two icons(8x8) after powerondownload
Effect for the above code
customized lamp for outdoor use
Connect and control with raspberry
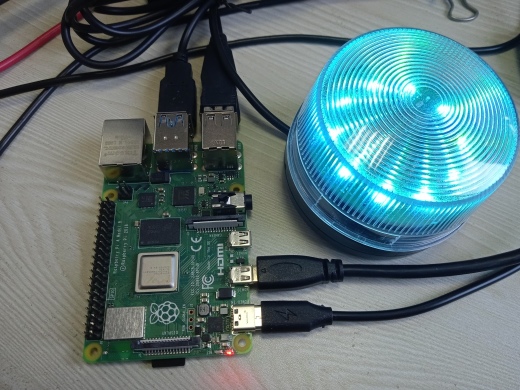
Single step debugging with raspberry
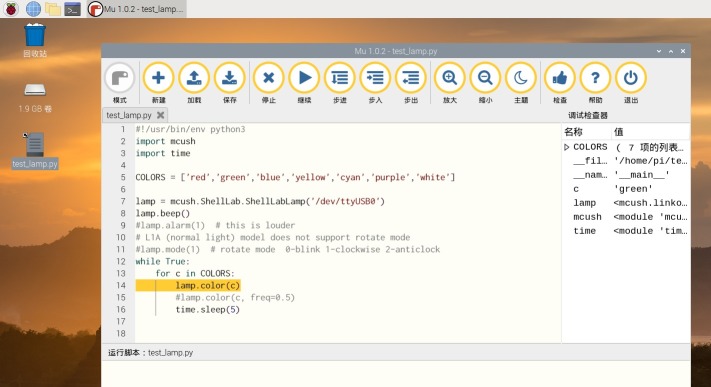
Control with Turtle Editor
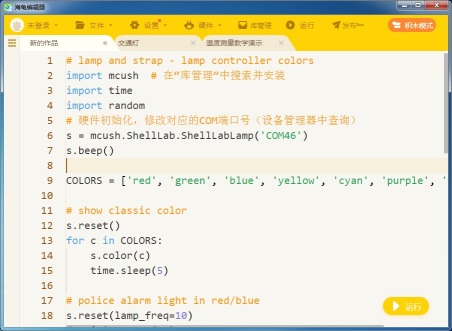
switch color alarm for collision detection in ROS
test with mcush_util
Multi color blinking
Long strip
Use push command
Push command (faster)
Push command (32*8=256 LEDs, rainbow waterfall)
Configured as RGBCMY loop after poweron download
|
Configured as police alarm flashdownload
|
C-API Compiled waterfall
|
Configured as a command-triggered waterfall stripdownload
|
Configured to switch between two icons(8x8) after powerondownload
|
customized lamp for outdoor use
Connect and control with raspberry
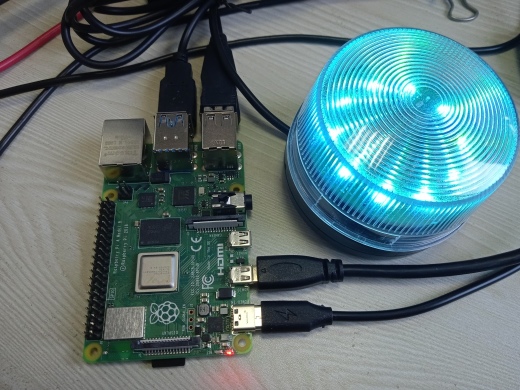
Single step debugging with raspberry
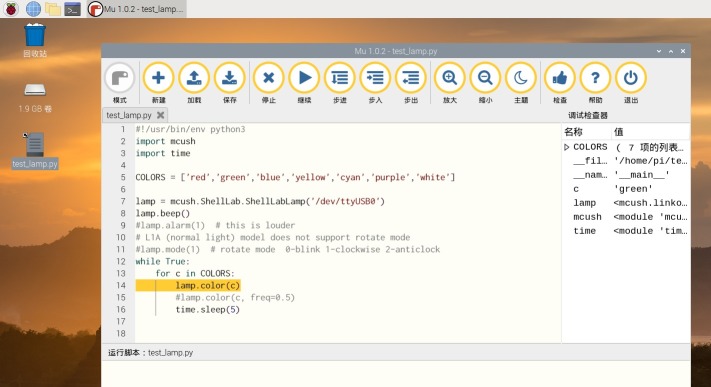
Control with Turtle Editor
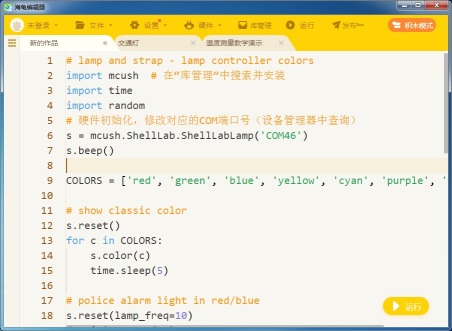
switch color alarm for collision detection in ROS
test with mcush_util
|