Hardware
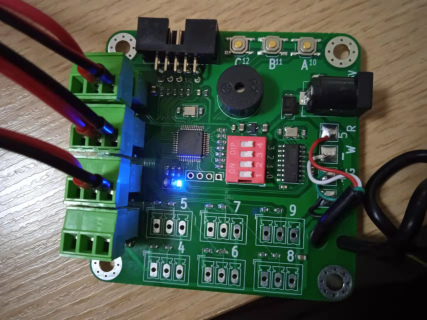
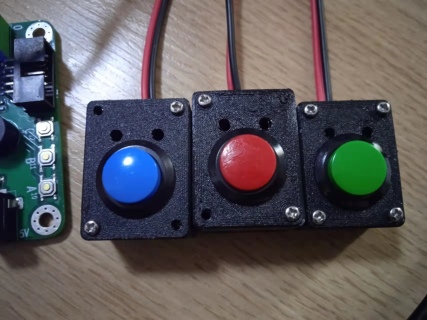
Shell Lab K1 is programmable key/switch controller Characteristic: ● 10 external key/switch inputs, 3 on-board keys● built-in software debounce algorithm with configurable parameters ● support event message (on/off/long press) with timestamp, queue buffered ● support enable/disable for each channel ● at startup, parameters are auto configured by mode switch ● expand channels from extend socket ● USB powered, 2-meter cable length ● interactive serial console with ASCII command, online manual embedded ● programming language is not limited (Python recommended) ● quick test with Testbench App (with various demo codes) ● pip install mcush library (support windows/linux/mac) and write scripts easily Use cases: ● manual intervention in step/cycle tests● experiment progress control ● circumstances that front-end control (HID keyboard) is forbidden and backend control is MUST ● interactive game design ● educational experiment design ● outdoor case, manual control ● system integration for industrial equipment ● product prototype design ● art creativity Customize: ● customized PCB size, channel number, input signal conditioning |
Software
Serial Communication FAQ C Programming FAQ Serial command: Key control command
Example: query all channel state
enable channel
disable channel
key input signal invert
query events buffer
software debounce algorithm
events statistics
Python API: Install: sudo pip3 install mcushUpgrade: sudo pip3 install -U mcush
Examples:
Download: Shell Lab Testbench Application |
Application
Built-in script demo
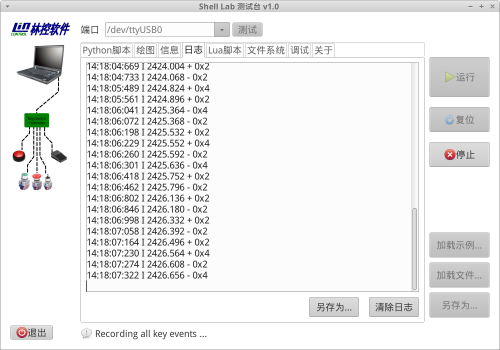
|
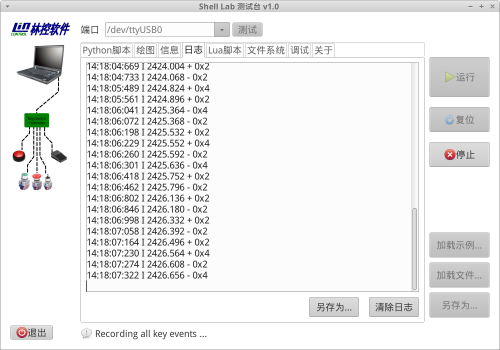