硬件
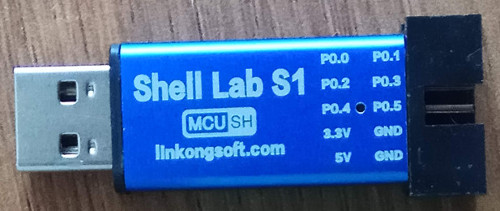
Shell Lab S1是USB接口精简版控制器。
控制器+接线端子
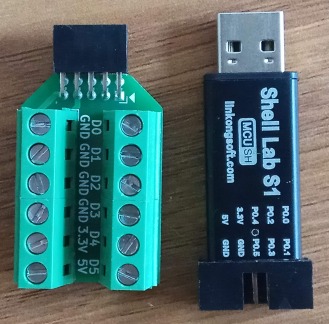
接线说明
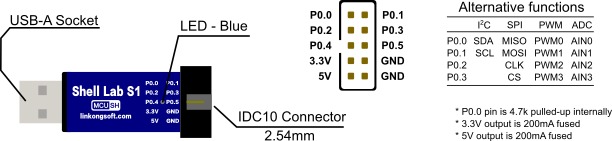
扩展模块: NPN-MOS扩展
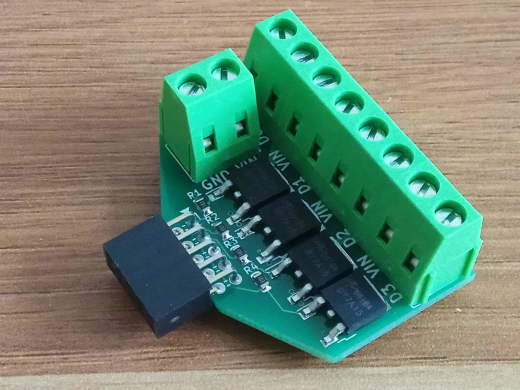
扩展模块: 继电器扩展
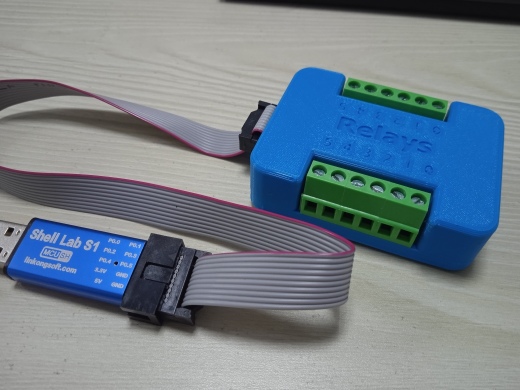
应用
继电器流水灯 下载
用C-API编译上述流水灯
用PWM控制直流电机软启停 下载
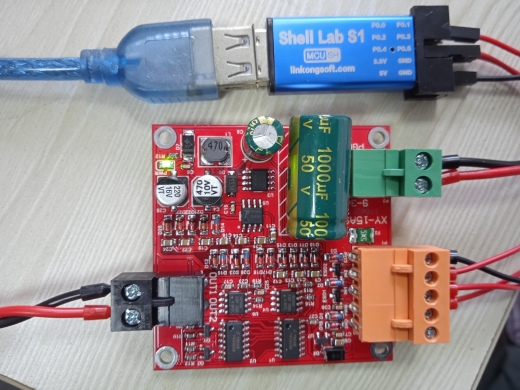
连接树莓派控制
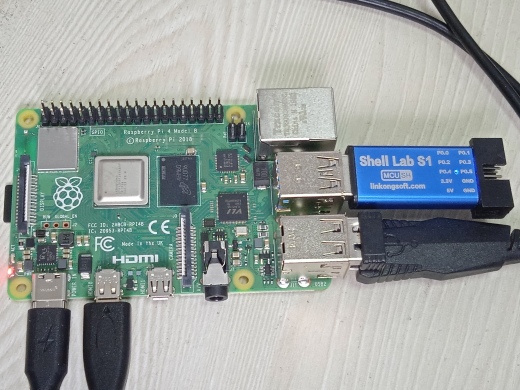
连接香橙派控制
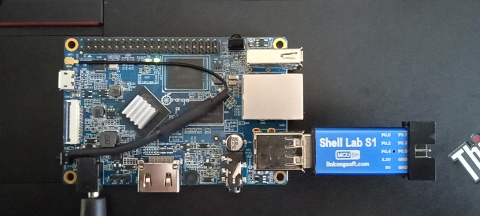
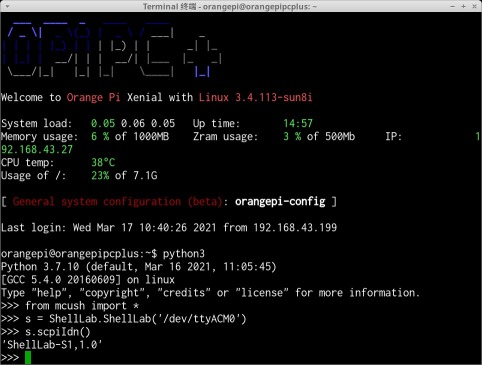
用mcush_util测试
|
用C-API编译上述流水灯
|
|
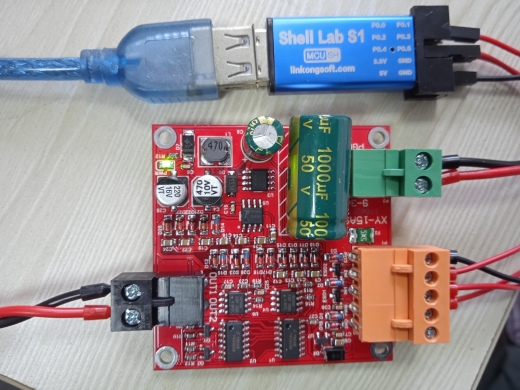
连接树莓派控制
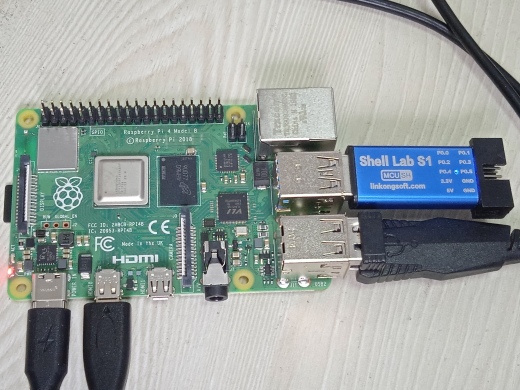
连接香橙派控制
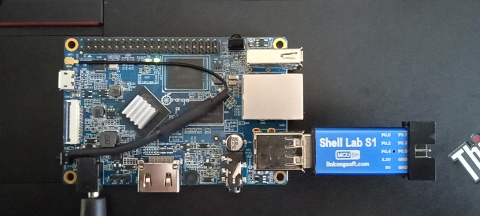
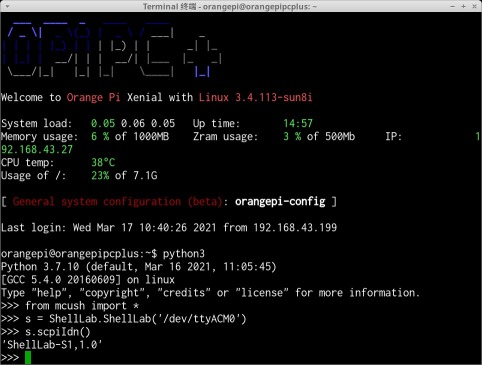
用mcush_util测试
|