Hardware
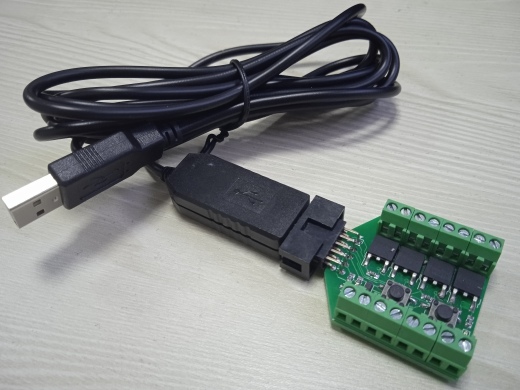
Shell Lab L4 is programmable LED spotlight / tower lamp controller with USB/serial interface Characteristic: ● 4 PWM modulated NPN-MOS to driver high power LED lamps● independently configurable between each channel: power, blink frequency, counter ● RGB each channel is independently adjusted from 0~255 ● blinking effect (freq adjustable from 0.1~20Hz, 0 for static) ● not limited to LED, other PWM modulated devices are available: DC-motors, heating wires... ● 2 meters length of USB cable ● interactive serial console with ASCII command, online manual embedded ● programming language is not limited (Python recommended) ● quick test with Testbench App (with various demo codes) ● pip install mcush library (support windows/linux/mac) and write scripts easily Use cases: ● prototype design● fault/exception indication in product/software cycle test ● indication in assembly line, green for PASS and red for FAIL ● experiment progress indication ● kanban/placard indication ● interactive game design ● educational experiment design ● outdoor case, auto indicator ● system integration for industrial equipment ● art creativity ● stage light control ● landscape light control ● DIY toy ● shop sign for advertisement Customize: ● customize PCB and case● customize firmware for module integration ● switch to bluetooth communication, for mobile control ● RS232 interfaced Attention: ● commands in lamp mode are very similar to L1 signal lamp, easy for replacement● NMOS driver board needs 12~24V DC power |
Software
Serial Communication FAQ C Programming FAQ Serial command:
Example: (after poweron and self-test, all channel outputs are disabled)set #0 lamp 100% power, no blink:
set #1 lamp 50% power, 5Hz, blink 10 cycles and set off:
cancel the cycle limit for #1, blinks forever:
set all channels synchronized
Python API: Install: sudo pip3 install mcushUpgrade: sudo pip3 install -U mcush
Examples:
Download: Shell Lab Testbench Application |
Application
Strips blink/breathe effect
Tower lamp blink effect
Configured as sensor/key triggered blink/beep alarm
Code effect
Tower lamp blink effect
Configured as sensor/key triggered blink/beep alarm
|