Hardware
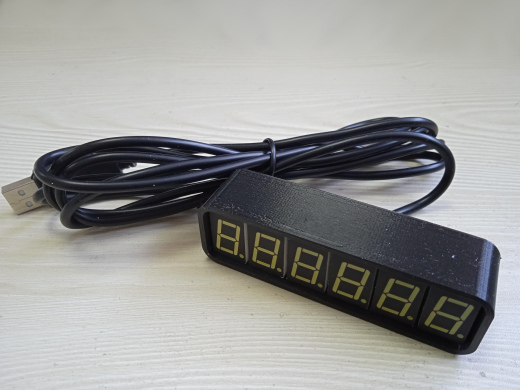
Shell Lab L3 is programmable 8-segments LED display with USB/serial interface Characteristic: ● 6-digital 0.56inch bright white 8-segment LED display● all segments configurable, support alphabet (portion) ● support blink mode ● black 3D-printed case ● USB powered, cable length 2-meter ● interactive serial console with ASCII command, online manual embedded ● programming language is not limited (Python recommended) ● quick test with Testbench App (with various demo codes) ● pip install mcush library (support windows/linux/mac) and write scripts easily Use cases: ● prototype design● in-progress value indication in product/software cycle test ● indication in assembly line, green for PASS and red for FAIL ● experiment progress indication ● kanban/placard indication ● logistic/storage management, goods location indicator ● interactive game design ● educational experiment design ● outdoor case, auto indicator ● system integration for industrial equipment Customize: ● other digits/size display |
Software
Serial Communication FAQ C Programming FAQ Serial command: display control command
Example: clear screen
display string "HELLO"
display string "3.14" from 2nd position
display number 9 at 4th position
clear char at 5th position
blink whole display:
set blink mode at 3rd positio
display integer 1234 (right aligned)
Python API: Install: sudo pip3 install mcushUpgrade: sudo pip3 install -U mcush
Example:
Download: Shell Lab Testbench Application |
Application
display demo
0.8 inch version
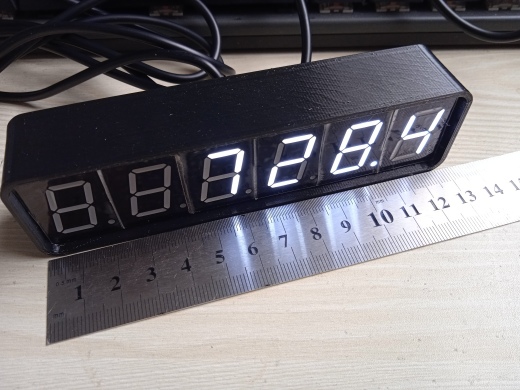
3 inch version
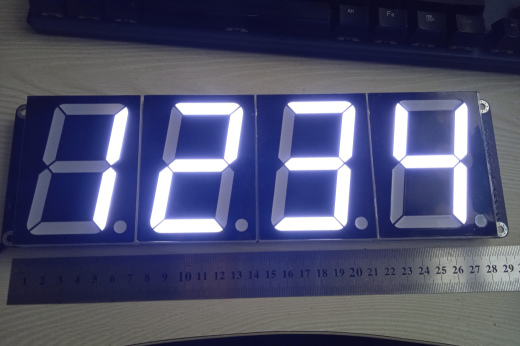
test with mcush_util
|
0.8 inch version
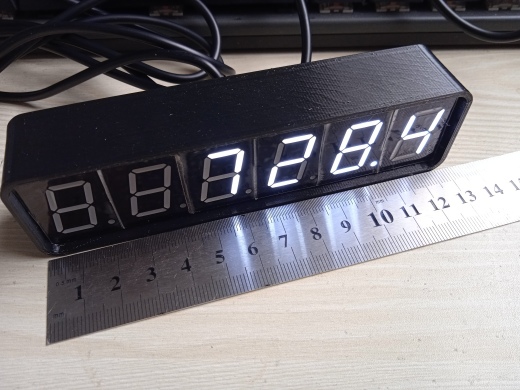
3 inch version
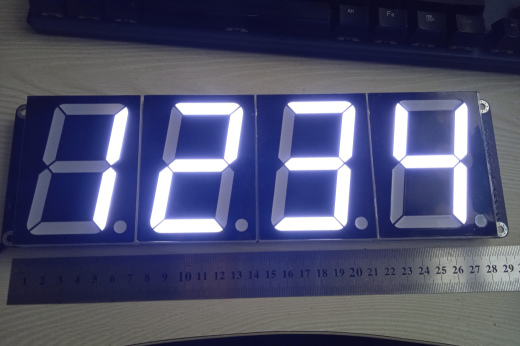
test with mcush_util
|