Hardware
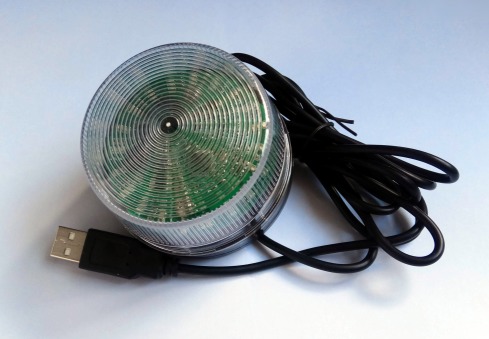
Shell Lab L1 is a programmable LED signal lamp with USB/serial interface Characteristic: ● PWM to adjust RGB brightness (each channel is independently adjusted from 0~255)● blinking effect (freq adjustable from 0.1~20Hz, 0 for static) ● L1B model(brighter), switch to WS2812 chip, double brightness ● L1B model(brighter), support rotation mode, current is stable ● beeper embedded, frequency/duration are adjustable, less volume ● alarm embedded (optional), louder volume, with adjustable down counter ● interactive serial console with ASCII command, online manual embedded ● programming language is not limited (Python recommended) ● quick test with Testbench App (with various demo codes) ● pip install mcush library (support windows/linux/mac) and write scripts easily Use cases: ● fault/exception indication in product/software cycle test● indication in assembly line, green for PASS and red for FAIL ● experiment progress indication ● kanban/placard indication ● logistic/storage management, goods location indicator ● ROS robot indication ● interactive game design ● educational experiment design ● outdoor case, auto indicator ● system integration for industrial equipment ● art creativity Work modes: ![]() Install: fix the accessory buckle buddy first, then pile the lamp and rotate a little![]() ![]() Customize: ● change USB interface to TTL● Bluetooth/serial convert embedded, for wireless control ● default 2-meter length cable, customizable Attention: ● long time working in static mode is not recommended because:worse indication effect and decrease in LED lifetime ● for multi-lamps condition, add extra power for USB-HUB |
Software
Serial Communication FAQ C Programming FAQ Serial command: 1. LED Control Command:
2. Beeper Control:
3. Alarm Control (louder):
Example: blink in RED color, 1Hz(default):
blink in GREEN color, 2Hz:
blink in BLUE color, 0.5Hz:
blink YELLOW color, 0.1Hz:
blink PURPLE color, 10Hz:
static CYAN color:
single RED channel as 64:
single GREEN channel as 0x80:
single BLUE channel as 0x200:
query current color/freq setting:
short beep @4kHz/50ms(default):
short beep @2kHz/1sec:
Alarm once @1Hz:
Alarm ten times @10Hz:
Alarm down counter and freq:
Rotation mode (L1B model only):
Python API: Install: sudo pip3 install mcushUpgrade: sudo pip3 install -U mcush
Example:
Download: Shell Lab Testbench Application |
Application
Single lamp blinking effect
Multi lamps blinking effect
Rotation mode for L1B model only
Enhanced version, 20 groups of LEDs for smooth brightness
Case and PCB customized
Deployed in headless NAS, use serial lamp to indicator remote connectiondownload
Config the lamp to looly display RGBCMY color after powerup download
Control with Raspberry board
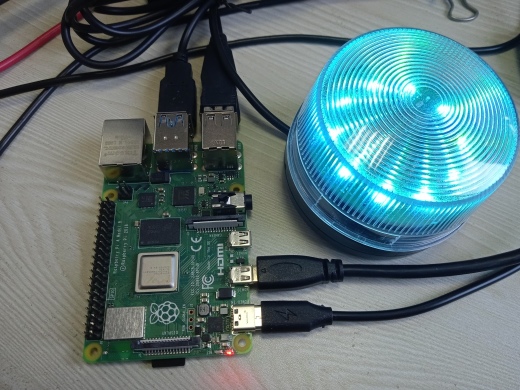
Single step debug with Raspberry board
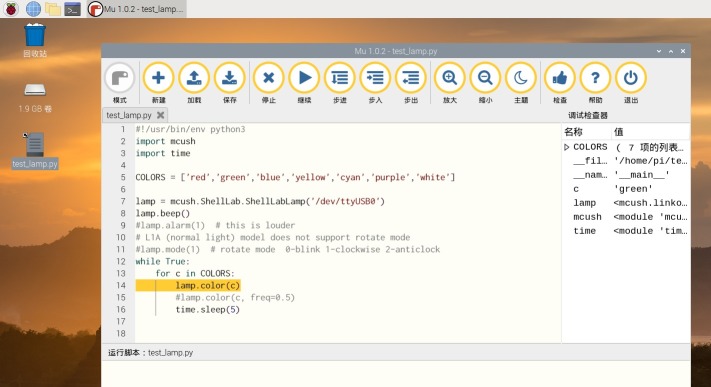
test with mcush_util
Multi lamps blinking effect
Rotation mode for L1B model only
Enhanced version, 20 groups of LEDs for smooth brightness
Case and PCB customized
Deployed in headless NAS, use serial lamp to indicator remote connectiondownload
|
Config the lamp to looly display RGBCMY color after powerup download
|
Control with Raspberry board
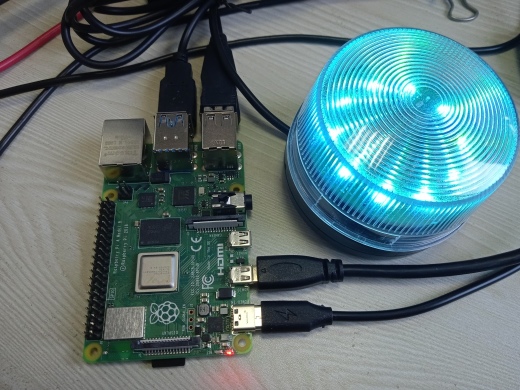
Single step debug with Raspberry board
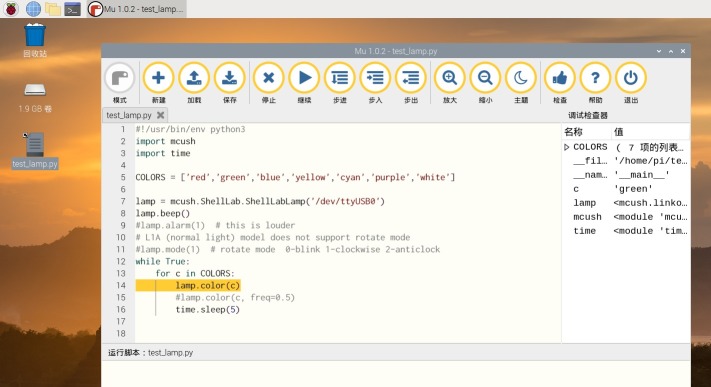
test with mcush_util
|